Lab 05
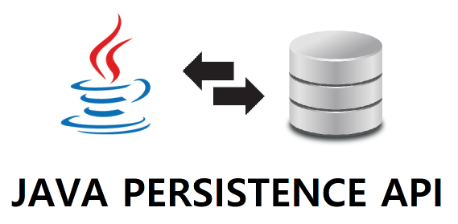
Library Lombok, java record
Java Persistent API
In project lab 1 , lab 2, lab 3
Modify the project to store Score in the database using JPA.
Dependencies
To use JPA we need to add dependencies to the jakarta.persistence-api library version 3.2.0 and hibernate-core version 6.6.11.Final
Module info
The project will need to use the jakarta.persistence and org.hibernate.orm.core modules.
Also, the lab.data package needs to be opened by everyone not just the javafx.base package to allowe Hibernate can process it using reflection.
JPA configuration
Add the persistence.xml file to the src/main/resources/META-INF folder. This file is main configuration file for the JPA.
The contents of the file are listed below and you can find the name of the persistence unit there (you will use in the source code), the database connection settings and the settings for the Hibernet, such as that Hibernate should try to create a database schema based on entity classes.
<?xml version="1.0" encoding="UTF-8"?>
<persistence version="3.0"
xmlns="https://jakarta.ee/xml/ns/persistence"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="
https://jakarta.ee/xml/ns/persistence
https://jakarta.ee/xml/ns/persistence/persistence_3_0.xsd">
<persistence-unit name="java2"
transaction-type="RESOURCE_LOCAL">
<!-- If you are running in a production environment, add a managed data
source, this example data source is just for development and testing! -->
<properties>
<property name="jakarta.persistence.jdbc.url"
value="jdbc:h2:file:./db/java2" />
<!-- In memory DB no store to disk
<property name="jakarta.persistence.jdbc.url" value="jdbc:h2:mem:java2" />
-->
<property name="jakarta.persistence.jdbc.driver"
value="org.h2.Driver" />
<property name="jakarta.persistence.jdbc.user" value="app" />
<property name="jakarta.persistence.jdbc.password"
value="app" />
<property
name="jakarta.persistence.schema-generation.database.action"
value="create"></property>
<!-- Properties for Hibernate -->
<property name="hibernate.show_sql" value="false" />
<property name="hibernate.format_sql" value="true" />
<!--
<property name="hibernate.hbm2ddl.auto" value="update" />
-->
</properties>
</persistence-unit>
</persistence>
Entity class Score
Modify the Score class to be a valid entity class:
- Add an annotation for the entity class
- Add an annotation for id and an annotation for automatic id generation
- Add a constructor without parameters
JpaConnector
Implement the JPA connector methods so that the game works as intended and passes all UnitTests.
- You need to create and store EntityManagerFactory and EntityManager. To do this, you could use a constructor.
- EntityManager can use createQuery to create a query very similar to SQL, but beware the differences (see lecture)
- For methods that change the DB content you have to use transactions (getTransaction and begin and commit)
- The save method should use the entity id to decide whether to use persist or merge and should should return the saved object.
- The modifyNoPersistOrMerge method should retrieve Score by id and then apply to it the passed Consumer to modify the score. It should then ensure that the data is synchronized with the DB, to save the data, but don't use the persist or merge methods, remember what we said in the lecture on managed entities.
Change in Score
Make sure that the enum value of level is stored in the DB as text and not as a number.
- Use an appropriate annotation
- Don't forget to delete the DB, it changes the column type and this is not automatic
Solution
Solution can be found https://gitlab.vsb.cz/jez04-vyuka/java2/labs/java2lab05v1/-/tree/solution?ref_type=heads in branche solution.
https://gitlab.vsb.cz/jez04-vyuka/java2/labs/java2lab05v2/-/tree/solution?ref_type=heads
https://gitlab.vsb.cz/jez04-vyuka/java2/labs/java2lab05v3/-/tree/solution?ref_type=heads